# ajax - PUT, PATCH, DELETE
jQuery ajax 의 HTTP METHOD가 PUT, PATCH, DELETE 일 때, @ResponseBody로 파라미터 매핑하기
# 환경
/**
* tool: STS 4.13.0
* version: 2.7.3-SNAPSHOT
* java: 1.8
* type: MAVEN
* view: THYMELEAF
* jQuery: 3.6.0
*/
# page
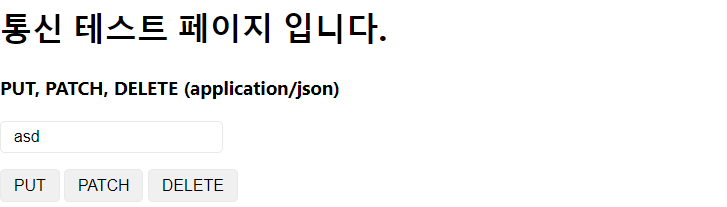
# html (일부분 발췌)
<h3>PUT, PATCH, DELETE (application/json)</h3>
<div>
<input type="text" id="requsetsNew" placeholder="Put, Delete Test" style="display: inline;"/>
<br>
<input type="button" onclick="test.requsetsNew('put')" value="PUT" style="display: inline;"/>
<input type="button" onclick="test.requsetsNew('patch')" value="PATCH" style="display: inline;"/>
<input type="button" onclick="test.requsetsNew('delete')" value="DELETE" style="display: inline;"/>
</div>
# function (일부분 발췌)
const test = {
requsetsNew: function(method) {
const input = {
param1: $('#requsetsNew').val(),
param2: method
}
sendRequestNew(`/test/send/${method}`, method, JSON.stringify(input),
function(result) {
console.log(result);
},
);
},
}
# jQuery ajax (일부분 발췌)
function sendRequestNew(url, method, input, cbSuccess) {
$.ajax({
// options
url : url,
method : method,
data : input,
contentType : 'application/json; charset=utf-8',
dataType : "json",
beforeSend : function(jqXHR, settings) {}
// success
}).done(function(output, textStatus, jqXHR) {
cbSuccess(output);
// error
}).fail(function(jqXHR) {
console.log("ERROR");
// complete
}).always(function(output, textStatus, jqXH) {
console.log("COMPLETE");
}
);
}
# @Controller (일부분 발췌)
@Slf4j
@Controller
@RequestMapping("/test/send")
public class TestSendController {
@PutMapping("/put")
public @ResponseBody Map<String, String> put(@RequestBody Map<String, String> input) {
log.debug("[PARAMETER] {}", input);
return input;
}
@PatchMapping("/patch")
public @ResponseBody Map<String, String> patch(@RequestBody Map<String, String> input) {
log.debug("[PARAMETER] {}", input);
return input;
}
@DeleteMapping("/delete")
public @ResponseBody Map<String, String> delete(@RequestBody Map<String, String> input) {
log.debug("[PARAMETER] {}", input);
return input;
}
}
# 내용
Controller의 메소드가 @PutMapping, @PatchMapping. @DeleteMapping 일 때, 제약이 있다.
- 브라우저는 http method가 PUT, PATCH, DELETE, ajax의 contentType이 application/x-www-form-urlencoded 일 경우, 파라미터를 파싱해주지 않는다.
- 이슈: https://softwareengineering.stackexchange.com/questions/114156/why-are-there-no-put-and-delete-methods-on-html-forms
- 위 와 같은 이유로 보통 GET, POST 만 사용,,,!
- GET, POST만 사용 가능할 때 PUT, PATCH, DELETE 사용하는 방법은 아래 참조 확인하세요!
# ajax 요청 방식에 따른 파라미터 맵핑 방법.
https://hjho95.tistory.com/14 ajax settings
https://hjho95.tistory.com/7 application/x-www-form-urlencoded
https://hjho95.tistory.com/8 application/json
https://hjho95.tistory.com/9 form tag
https://hjho95.tistory.com/10 multipart/form-data
https://hjho95.tistory.com/12 PUT, PATCH, DELETE
https://hjho95.tistory.com/11 POST 방식으로 PUT, PATCH, DELETE 사용하기
# ajax 시리즈의 참조 페이지.
ajax settings: https://api.jquery.com/jquery.ajax/#jQuery-ajax-url-settings
@RequestBody: https://docs.spring.io/spring-framework/docs/current/javadoc-api/org/springframework/web/bind/annotation/RequestBody.html
'스프링 부트' 카테고리의 다른 글
[SpringBoot] jdk 1.8 > jdk 11 로 변경하기 (2) | 2022.09.12 |
---|---|
[SpringBoot] ajax - POST방식으로 PUT, PATCH, DELETE 사용하기! (HiddenHttpMethodFilter) (0) | 2022.08.15 |
[SpringBoot] ajax - multipart/form-data 로 요청하기! (0) | 2022.08.14 |
[SpringBoot] ajax - html form tag 로 요청하기! (0) | 2022.08.13 |
[SpringBoot] ajax - application/json 로 요청하기! (0) | 2022.08.13 |