# fetch - POST, application/json
주로 사용하는 ajax 대신 fetch 로 POST방식 application/json 타입으로 통신하기!
# 환경
/**
* tool: sts 4.13.0
* version: 2.7.3-SNAPSHOT
* java: 1.8
* type: maven
* view: Thymeleaf
*/
# test page
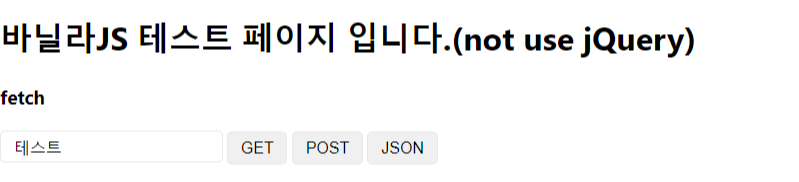
# test page html
<h1>바닐라JS 테스트 페이지 입니다.(not use jQuery)</h1>
<h3>fetch</h3>
<input type="text" id="reqValue" value="테스트" style="display: inline;"/>
<input type="button" onclick="get()" value="GET" style="display: inline;"/>
<input type="button" onclick="post()" value="POST" style="display: inline;"/>
<input type="button" onclick="json()" value="JSON" style="display: inline;"/>
# fetch (json) javascript example code
function json() {
// const value = document.getElementById('reqValue').value;
const params = {
text: reqValue.value,
number: 2001
}
fetchJson("/test/vanilla/fetch/json", params)
.then((data) => {
// JSON 데이터가 `data.json()` 호출에 의해 파싱됨
console.log('OUTPUT:', data.type, data.result,);
}).catch((error) => {
console.log('ERROR:', error.message);
}
);
}
// JSON POST 메서드 구현 예제
async function fetchJson(uri, params) {
const data = JSON.stringify(params);
console.log('INPUT:', data);
const header = document.querySelector("meta[name='_csrf_header']").attributes.content.value;
const token = document.querySelector("meta[name='_csrf']").attributes.content.value;
const headers = new Headers();
headers.append('Content-Type', 'application/json; charset=UTF-8');
headers.append(header, token);
// 옵션 기본 값은 *로 강조
const response = await fetch(uri, {
method: 'POST', // *GET, POST, PUT, DELETE 등
mode: 'cors', // no-cors, *cors, same-origin
cache: 'no-cache', // *default, no-cache, reload, force-cache, only-if-cached
credentials: 'same-origin', // include, *same-origin, omit
headers: headers,
redirect: 'follow', // manual, *follow, error
referrerPolicy: 'no-referrer', // no-referrer, *no-referrer-when-downgrade, origin, origin-when-cross-origin, same-origin, strict-origin, strict-origin-when-cross-origin, unsafe-url
body: data, // body의 데이터 유형은 반드시 "Content-Type" 헤더와 일치해야 함
});
if(!response.ok) {
let message = '';
switch(response.status) {
case 403: message = '권한이 없습니다.'; break;
case 404: message = 'URI가 올바르지 않습니다.'; break;
default: message = '네트워크 응답이 올바르지 않습니다.'; break;
}
throw new Error(message);
}
return response.json(); // JSON 응답을 네이티브 JavaScript 객체로 파싱
}
- header, token은 제외해도 됨.
- Security Config + csrf 활성화 상태라서 작성한 코드.
# fetch (json) java example code
@Slf4j
@Controller
@RequestMapping("/test/vanilla")
public class TestVanillaController {
@PostMapping("/fetch/json")
public ModelAndView fetchJson(@RequestBody Map<String, Object> input) {
log.debug("[PARAMETER] {}", input);
ModelAndView mav = new ModelAndView("jsonView");
mav.addObject("result", input);
mav.addObject("type", "post-json");
return mav;
}
}
# log
javascript log
INPUT: {"text":"테스트","number":2001}
OUTPUT: post-json {number: 2001, text: '테스트'}
java log
[PARAMETER] {number=2001, text=테스트}
# Vanilla JS 시리즈
https://hjho95.tistory.com/18 fetch - GET, application/x-www-form-urlencoded
https://hjho95.tistory.com/19 fetch - POST, application/x-www-form-urlencoded
https://hjho95.tistory.com/20 fetch - POST, application/json
# Vanilla JS 시리즈의 참조 페이지.
MDN fetch: https://developer.mozilla.org/ko/docs/Web/API/Fetch_API/Using_Fetch
'자바스크립트' 카테고리의 다른 글
[JavaScript] async, await를 이용한 ajax 통신 (0) | 2022.09.10 |
---|---|
[JavaScript] async, await 테스트 (2) | 2022.09.10 |
[Vanilla JS] fetch - POST, application/x-www-form-urlencoded 로 요청하기! (0) | 2022.09.04 |
[Vanilla JS] fetch - GET, application/x-www-form-urlencoded 로 요청하기! (0) | 2022.09.04 |
[Vanilla JS] Hoisting, var, let, const 정리 (0) | 2022.09.04 |